Q. Write a function in to search and display details of all trains, whose destination is Delhi from a binary file TRAIN.DAT. Assuming the binary file is containing the objects of the following dictionary type:
Train = { 'Tno': ___ , 'From': ____ , 'To' : ____}
You can understand by Watching video :-
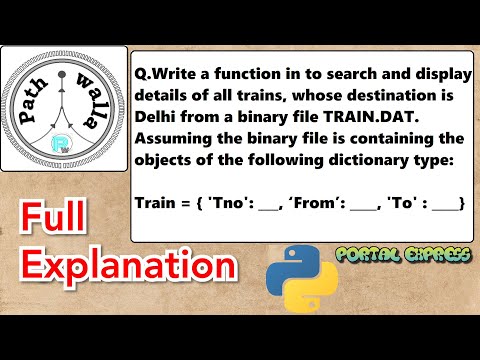
Answer :-
import pickle def search() : file = open("TRAIN.DAT","rb") found = 0 try : while True : Train = pickle.load(file) if Train [ "To" ] == 'Delhi': print(Train) found=1 except EOFError : if found == 0: print("Not found !!!") file.close() search()
Program for writing in the binary file :-
import pickle f = open("TRAIN.DAT","wb") while True : Tno = int(input("Enter Train number ( -1 for exit ) :- ")) if Tno == -1 : break From = input("Enter Station name :- ") To = input("Enter Station name where that train will go:- ") Train = { 'Tno': Tno , 'From': From , 'To' : To } pickle.dump( Train , f) print("Your file is ready.") f.close()
The output of the above program :-
Enter Train number ( -1 for exit ) :- 456365
Enter Station name :- Kota
Enter the Station name where that train will go:- Mub
Enter Train number ( -1 for exit ) :- 53764
Enter Station name :- Kota
Enter the Station name where that train will go:- Delhi
Enter Train number ( -1 for exit ) :- 45343
Enter Station name :- Pray
Enter the Station name where that train will go:- JK
Enter Train number ( -1 for exit ) :- 34356
Enter Station name :- Delhi
Enter the Station name where that train will go:- Mub
Enter Train number ( -1 for exit ) :- 93641
Enter Station name :- JK
Enter the Station name where that train will go:- Delhi
Enter Train number ( -1 for exit ) :- 63444
Enter Station name :- Mub
Enter the Station name where that train will go:- Delhi
Enter Train number ( -1 for exit ) :- -1
Your file is ready.
>>>
Enter Station name :- Kota
Enter the Station name where that train will go:- Mub
Enter Train number ( -1 for exit ) :- 53764
Enter Station name :- Kota
Enter the Station name where that train will go:- Delhi
Enter Train number ( -1 for exit ) :- 45343
Enter Station name :- Pray
Enter the Station name where that train will go:- JK
Enter Train number ( -1 for exit ) :- 34356
Enter Station name :- Delhi
Enter the Station name where that train will go:- Mub
Enter Train number ( -1 for exit ) :- 93641
Enter Station name :- JK
Enter the Station name where that train will go:- Delhi
Enter Train number ( -1 for exit ) :- 63444
Enter Station name :- Mub
Enter the Station name where that train will go:- Delhi
Enter Train number ( -1 for exit ) :- -1
Your file is ready.
>>>
The output of Main progarm :-
{'Tno': 53764, 'From': 'Kota', 'To': 'Delhi'}
{'Tno': 93641, 'From': 'JK', 'To': 'Delhi'}
{'Tno': 63444, 'From': 'Mub', 'To': 'Delhi'}
>>>
{'Tno': 93641, 'From': 'JK', 'To': 'Delhi'}
{'Tno': 63444, 'From': 'Mub', 'To': 'Delhi'}
>>>
full explain please
ReplyDeleteWatch the video dude
DeleteYes.
DeletePost a Comment
You can help us by Clicking on ads. ^_^
Please do not send spam comment : )