Q. Write a program to enter the following records in a binary file:
Item No integer
Item_Name string
Qty integer
Price float
Number of records to be entered should be accepted from the user.
Read the file to display the records in the following format:
Item No:
Item Name :
Quantity:
Price per item:
Amount: ( to be calculated as Price * Qty)
You can understand by Watching video :-
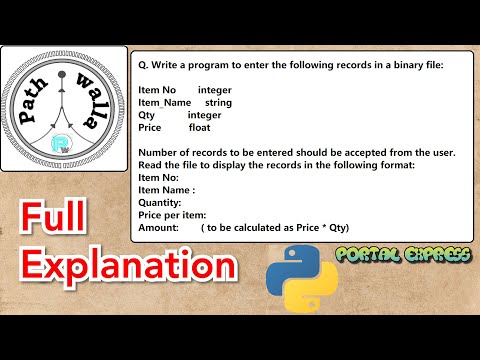
Answer :-
import pickle file = open("Pathwalla.dat","wb") while True : dic = { } Item_No = int(input("Enter Item no.")) Item_Name = input("Enter Item name :-") Qty = int(input("Enter Quantity :- ")) Price = float(input("Enter price :-")) dic[ "Item_No" ] = Item_No dic[ "Item_Name" ] = Item_Name dic[ "Qty" ] = Qty dic[ "Price" ] = Price pickle.dump( dic , file ) print() ans = input("Do you want to quit Enter [ Y/N ] :-") if ans == "y" or ans == "Y" : break print() file.close() print() file = open("Pathwalla.dat","rb") try : while True : dic = pickle.load(file) print( "Item No. :","\t", dic[ "Item_No" ] ) print( "Item Name :","\t", dic[ "Item_Name" ] ) print( "Quantity :","\t", dic[ "Qty" ] ) print( "Price of one :","\t",dic[ "Price" ] ) print( "Amount :", "\t" , dic[ "Qty" ] * dic[ "Price" ] ) print() except : file.close()
Output :-
Enter Item no.3544
Enter Item name :-Path
Enter Quantity :- 74
Enter price :-48.23
Enter Item name :-Path
Enter Quantity :- 74
Enter price :-48.23
Do you want to quit Enter [ Y/N ] :-n
Enter Item no.49978
Enter Item name :-Walla
Enter Quantity :- 74
Enter price :-12.65
Enter Quantity :- 74
Enter price :-12.65
Do you want to quit Enter [ Y/N ] :-n
Enter Item no.5432
Enter Item name :-Portal
Enter Quantity :- 98
Enter price :-18.46
Do you want to quit Enter [ Y/N ] :-n
Enter Item no.65654
Enter Item name :-Express
Enter Quantity :- 65496
Enter price :-0.59
Do you want to quit Enter [ Y/N ] :-y
Item No. : 3544
Item Name : Path
Quantity : 74
Price of one : 48.23
Amount : 3569.02
Item No. : 49978
Item Name : Walla
Quantity : 74
Price of one : 12.65
Amount : 936.1
Item No. : 5432
Item Name : Portal
Quantity : 98
Price of one : 18.46
Amount : 1809.0800000000002
Item No. : 65654
Item Name : Express
Quantity : 65496
Price of one : 0.59
Amount : 38642.64
>>>
Post a Comment
You can help us by Clicking on ads. ^_^
Please do not send spam comment : )